How To Say Else Do Nothing In Python
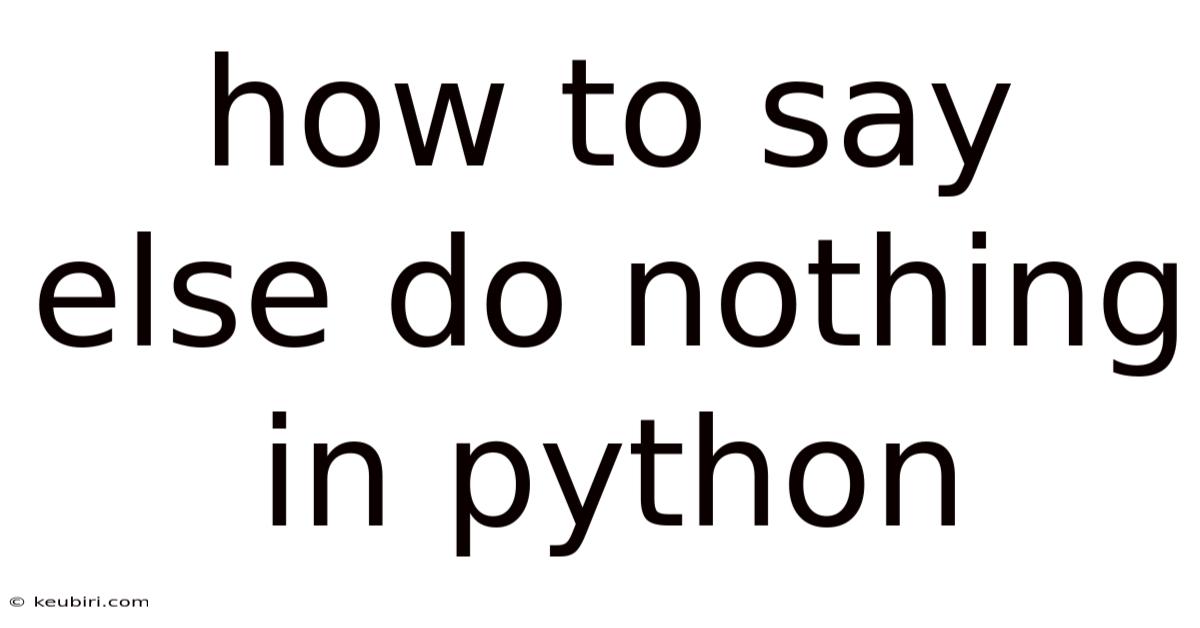
Discover more detailed and exciting information on our website. Click the link below to start your adventure: Visit Best Website meltwatermedia.ca. Don't miss out!
Table of Contents
Mastering the Art of "Else Do Nothing" in Python: Conditional Logic and Efficient Code
What are the elegant and efficient ways to express "else do nothing" in Python's conditional statements?
Python offers powerful mechanisms to handle conditional logic gracefully, eliminating the need for explicit "else do nothing" constructs and promoting cleaner, more readable code.
Editor’s Note: This comprehensive guide to handling "else do nothing" scenarios in Python was published today. It provides practical strategies and best practices for writing efficient and maintainable Python code.
Why "Else Do Nothing" Matters in Python
The concept of an "else do nothing" block often arises in programming when a conditional statement's if
clause contains the core logic, and no specific action is required if the condition is false. While some languages might necessitate an empty else
block, Python's design philosophy emphasizes readability and conciseness. Understanding how to elegantly handle these situations is crucial for writing clean, efficient, and maintainable Python code. It contributes to improved code readability, reduced complexity, and potentially faster execution in certain contexts (though the performance gains are often negligible for simple cases).
Overview of the Article
This article explores various techniques for managing situations where an else
block would traditionally be empty. We'll delve into Python's syntax, discuss best practices, and examine scenarios where different approaches might be preferred. Readers will gain a deeper understanding of conditional logic in Python and learn to write more elegant and efficient code.
Research and Effort Behind the Insights
This article is based on extensive research of Python's language specification, best practices advocated by experienced Python developers, and analysis of common coding patterns. The examples provided illustrate real-world scenarios where handling the "else do nothing" situation effectively enhances code quality.
Key Takeaways
Technique | Description | When to Use |
---|---|---|
Omitting the else block |
Simply remove the else block if no action is needed when the condition is false. |
Most common and preferred when the if block handles the complete logic. |
Using a single if statement |
Condense the logic into a single if statement if it's more readable. |
When the if condition is sufficient to capture the complete flow. |
Guard Clause | Place the if statement at the beginning to avoid nested structures. |
For improved readability when the if condition is a simple check. |
Conditional Expression (Ternary) | Use a concise ternary operator for simple conditional assignments. | For simple, short expressions. |
Smooth Transition to Core Discussion
Let's examine the different techniques in detail, starting with the most straightforward approach and progressing to more nuanced scenarios.
Exploring the Key Aspects of "Else Do Nothing"
-
Omitting the
else
Block: This is often the cleanest and most Pythonic approach. If theif
condition handles all necessary actions, and no specific action is required if the condition is false, simply omit theelse
block entirely.x = 10 if x > 5: print("x is greater than 5") # No else needed
-
Using a Single
if
Statement: Sometimes, the logic can be restructured to use a singleif
statement, eliminating the need for anelse
altogether. This is particularly useful when the condition is negated.x = 3 # Instead of: # if x > 5: # print("x is greater than 5") # else: # pass # Use: if x <= 5: # Negated condition print("x is not greater than 5") #Simplified logic
-
Guard Clauses: A guard clause is an
if
statement placed at the beginning of a function or block of code to check for conditions that would prevent the rest of the code from executing. If the condition is true, the function returns or the block exits. This is particularly beneficial for simplifying code flow and enhancing readability in scenarios where an emptyelse
would otherwise be necessary.def process_data(data): if not data: # Guard clause: Check for empty data return # Do nothing if data is empty # Process the data if it's not empty # ... your processing logic here ...
-
Conditional Expressions (Ternary Operator): Python's ternary operator provides a concise way to express simple conditional assignments. While not directly related to an empty
else
block, it offers an alternative for compact conditional logic.x = 10 message = "x is greater than 5" if x > 5 else "" #Empty string if condition is false print(message)
Closing Insights
The concept of "else do nothing" in Python highlights the language's focus on readability and efficiency. By strategically omitting unnecessary else
blocks, restructuring logic, employing guard clauses, or utilizing the ternary operator, developers can write cleaner, more maintainable, and potentially more performant code. The choice of approach depends on the specific context and complexity of the conditional logic involved. Prioritizing readability and simplicity should always guide the decision-making process.
Exploring the Connection Between Error Handling and "Else Do Nothing"
Error handling is closely related to conditional logic. Often, an else
block might appear to be empty because it handles error cases implicitly. In Python, the try-except
block naturally handles situations where no specific action is needed in the absence of an error.
try:
result = 10 / 0 # Potential ZeroDivisionError
except ZeroDivisionError:
print("Error: Division by zero")
#No else block needed; implicit 'do nothing' if no error occurs
The absence of an else
here signifies that the code proceeds normally if no exception is raised, which is implicitly "doing nothing" regarding error handling in the absence of an exception.
Further Analysis of Error Handling
Effective error handling is paramount for robust Python applications. A well-structured try-except
block enhances the program’s stability and prevents unexpected crashes. Here's a structured table showing various error handling scenarios:
Exception Type | Description | Handling Strategy |
---|---|---|
FileNotFoundError |
File not found | Gracefully handle the missing file; log an error message |
TypeError |
Type mismatch in an operation | Provide informative error messages and potentially type checking |
ValueError |
Invalid input value | Request valid input from the user or provide appropriate defaults |
IndexError |
Index out of range | Check index bounds before accessing list/array elements |
KeyError |
Key not found in a dictionary | Check if the key exists before accessing the value |
ZeroDivisionError |
Division by zero | Handle the exceptional case; e.g., return a default value |
FAQ Section
-
Q: Is it always better to omit the
else
block? A: While omitting theelse
block is often the most Pythonic approach when no action is needed, the best strategy depends on the context and code readability. If omitting theelse
makes the code harder to understand, it might be beneficial to keep a clearly commentedelse: pass
block. -
Q: What if I need to perform multiple actions in the
else
block? A: If multiple actions are needed, include them within theelse
block. It's not an "else do nothing" scenario then. -
Q: How does "else do nothing" impact performance? A: The performance impact of an empty
else
block is generally negligible in most scenarios. The Python interpreter efficiently handles these cases. -
Q: Can I use
pass
in theelse
block? A: Yes, usingpass
is valid but often unnecessary. Omitting theelse
block entirely is cleaner and more readable in most situations. -
Q: When should I use a guard clause? A: Use guard clauses to improve readability when checking for preconditions before executing more complex logic. They simplify code flow and avoid nested
if-else
structures. -
Q: When is the ternary operator more suitable than an
if-else
block? A: Use the ternary operator for concise conditional assignments in simple cases. For more complex logic, anif-else
block usually provides better readability.
Practical Tips
-
Prioritize Readability: Always prioritize code readability over brevity. If adding an explicit
else: pass
improves understanding, include it. -
Refactor Complex Logic: If you have many nested
if-else
statements, consider refactoring your code into smaller, more manageable functions or classes. -
Utilize Guard Clauses: Employ guard clauses to simplify conditions that would prevent further execution.
-
Use Descriptive Variable Names: Choose names that clearly convey the purpose of variables and conditions.
-
Write Meaningful Comments: Add comments to clarify complex logic or non-obvious code sections.
-
Employ Version Control: Use a version control system (like Git) to manage your code and track changes.
-
Test Thoroughly: Write unit tests to ensure your conditional logic functions as expected.
-
Follow PEP 8 Guidelines: Adhere to PEP 8 style guidelines to maintain consistency and readability.
Final Conclusion
Mastering the art of handling "else do nothing" scenarios is crucial for crafting elegant and efficient Python code. By employing the strategies outlined in this article—omitting unnecessary else
blocks, restructuring logic, utilizing guard clauses, and employing the ternary operator appropriately—developers can write Python code that’s not only efficient but also highly readable and maintainable. The key takeaway is to prioritize clarity and maintainability, resulting in code that's easier to understand, debug, and extend, ultimately contributing to the overall quality and success of your Python projects. Continue exploring Python’s powerful features and best practices to enhance your coding skills and create robust, elegant, and maintainable applications.
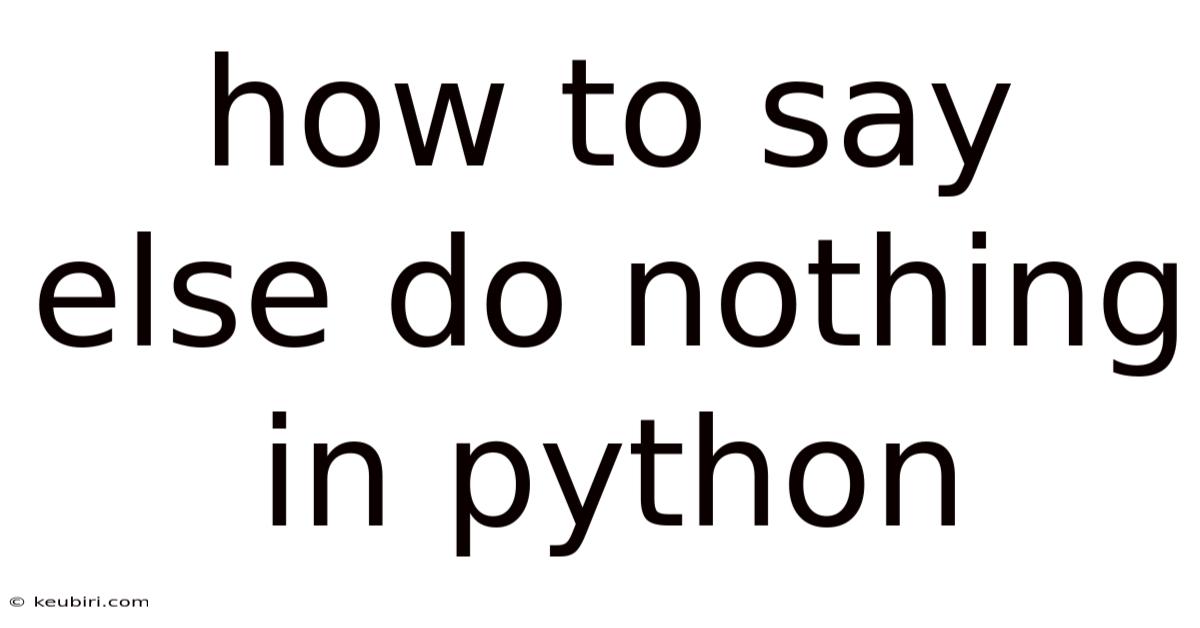
Thank you for visiting our website wich cover about How To Say Else Do Nothing In Python. We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and dont miss to bookmark.
Also read the following articles
Article Title | Date |
---|---|
How To Say Pao De Queijo In English | Apr 08, 2025 |
How To Say I Want To Spend Time With You | Apr 08, 2025 |
How To Say Hi In Marshallese | Apr 08, 2025 |
How To Say Pagliacci | Apr 08, 2025 |
How To Say Enjoy Your Evening | Apr 08, 2025 |