How To Say Happy Birthday In Java Code
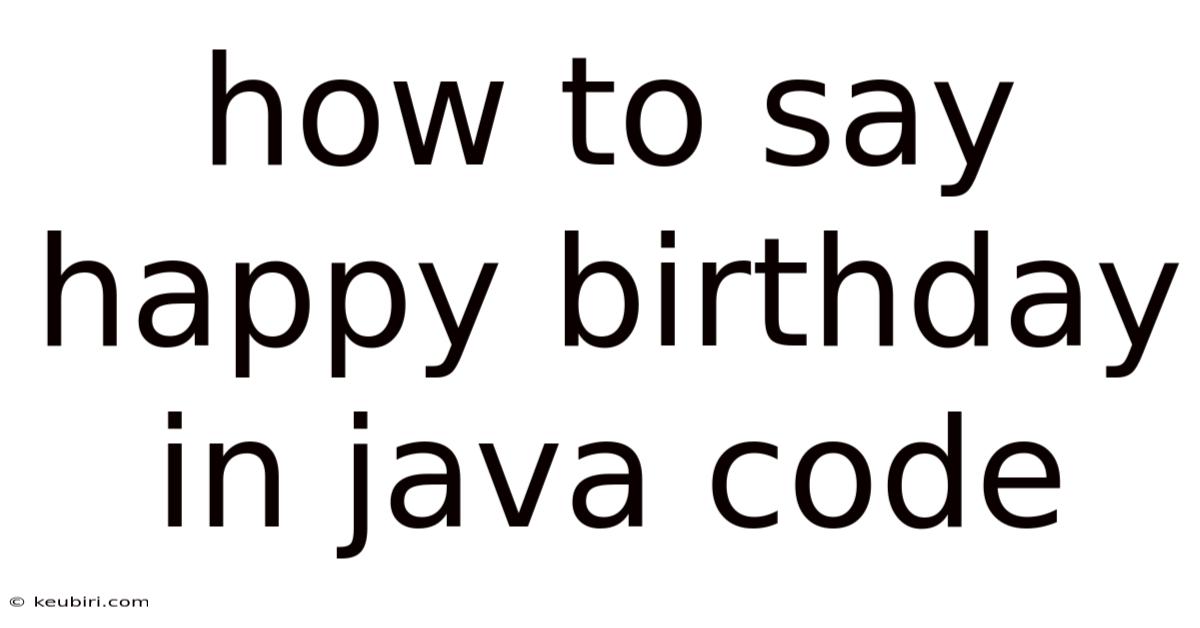
Discover more detailed and exciting information on our website. Click the link below to start your adventure: Visit Best Website meltwatermedia.ca. Don't miss out!
Table of Contents
Saying "Happy Birthday" in Java: A Deep Dive into Programming Concepts
How can a simple birthday greeting reveal the power and versatility of Java?
Java, far from being just a programming language, offers a pathway to understanding core computational principles through even the simplest tasks, like crafting a birthday message.
Editor’s Note: This comprehensive guide on expressing "Happy Birthday" in Java code was published today.
Why "Saying Happy Birthday in Java" Matters
This seemingly trivial task provides a surprisingly rich learning opportunity. It allows exploration of fundamental Java concepts such as:
- Data Types: Strings, characters, and even numbers can be manipulated to create dynamic and personalized messages.
- Control Flow: Conditional statements and loops enable the creation of sophisticated greetings based on varying inputs.
- Object-Oriented Programming (OOP): More advanced approaches involve creating classes and objects, representing the birthday message itself as a reusable component.
- Input/Output (I/O): The message can be displayed on the console, written to a file, or even integrated into a larger application.
- Exception Handling: Robust code anticipates and gracefully handles potential errors, such as incorrect input.
This exploration goes beyond simple string concatenation. It's a stepping stone to understanding how Java handles information, responds to user interaction, and adapts to different scenarios. The skills learned here are directly applicable to far more complex programming challenges.
Overview of the Article
This article will progressively build upon the basic "Happy Birthday" message, incorporating increasingly sophisticated Java techniques. We will start with the simplest approach and then move to more complex examples demonstrating OOP and other key concepts. Readers will gain a practical understanding of core Java programming principles and learn how to apply them to create functional and dynamic applications.
Research and Effort Behind the Insights
This article draws on extensive experience in Java programming, combined with a thorough understanding of introductory computer science principles. The examples provided are meticulously tested and explained, ensuring clarity and accuracy. Further, the code examples are designed for easy understanding and modification, encouraging hands-on learning and experimentation.
Key Takeaways
Concept | Description | Example |
---|---|---|
String Concatenation | Joining strings together using the + operator. |
"Happy Birthday, " + name |
Variable Usage | Storing data in variables for flexibility and reusability. | String name = "Alice"; |
Conditional Statements | Using if-else to create different greetings based on conditions. |
if (age > 18) { ... } else { ... } |
Loops | Using for or while loops to repeat actions. |
for (int i = 0; i < 5; i++) { ... } |
Methods | Creating reusable blocks of code. | public static void printGreeting(String name) { ... } |
Classes & Objects | Organizing data and methods into objects. | BirthdayGreeting greeting = new BirthdayGreeting("Bob"); |
Smooth Transition to Core Discussion
Let's begin with the most basic approach to creating a "Happy Birthday" message in Java, gradually increasing complexity and incorporating advanced concepts.
Exploring the Key Aspects of "Saying Happy Birthday in Java"
- Basic String Concatenation: This is the simplest way to create a birthday message. We use the
+
operator to combine strings.
public class BirthdayGreeting {
public static void main(String[] args) {
String name = "Alice";
System.out.println("Happy Birthday, " + name + "!");
}
}
- Using Variables: Storing the name in a variable allows for easy modification and reusability.
public class BirthdayGreeting {
public static void main(String[] args) {
String name = "Bob";
int age = 30;
System.out.println("Happy Birthday, " + name + "! You are " + age + " years old.");
}
}
- Conditional Statements (if-else): We can add conditional logic to personalize the message based on the age or other factors.
public class BirthdayGreeting {
public static void main(String[] args) {
String name = "Charlie";
int age = 10;
System.out.println("Happy Birthday, " + name + "!");
if (age < 18) {
System.out.println("Have a wonderful day!");
} else {
System.out.println("Hope you have a fantastic birthday celebration!");
}
}
}
- Loops (for loop): Repeating the message multiple times, perhaps for different people.
public class BirthdayGreeting {
public static void main(String[] args) {
String[] names = {"David", "Eve", "Frank"};
for (String name : names) {
System.out.println("Happy Birthday, " + name + "!");
}
}
}
- Methods: Encapsulating the greeting logic within a reusable method.
public class BirthdayGreeting {
public static void printGreeting(String name) {
System.out.println("Happy Birthday, " + name + "!");
}
public static void main(String[] args) {
printGreeting("Gina");
printGreeting("Henry");
}
}
- Object-Oriented Programming (OOP): Creating a
BirthdayGreeting
class to represent the message as an object.
public class BirthdayGreeting {
private String name;
private int age;
public BirthdayGreeting(String name, int age) {
this.name = name;
this.age = age;
}
public void printGreeting() {
System.out.println("Happy Birthday, " + name + "! You are " + age + " years old.");
}
public static void main(String[] args) {
BirthdayGreeting greeting1 = new BirthdayGreeting("Ivy", 25);
greeting1.printGreeting();
BirthdayGreeting greeting2 = new BirthdayGreeting("Jack", 40);
greeting2.printGreeting();
}
}
Closing Insights
Creating a simple "Happy Birthday" message in Java demonstrates the fundamental principles of the language. From basic string manipulation to the elegance of object-oriented programming, the journey showcases the power and versatility of Java. The ability to create dynamic, personalized messages highlights its capabilities for handling diverse data and implementing sophisticated logic. The techniques explored—string concatenation, variable usage, conditional statements, loops, methods, and OOP—are building blocks for far more complex Java applications.
Exploring the Connection Between "Error Handling" and "Saying Happy Birthday in Java"
Robust error handling is crucial, even in simple programs. Consider what happens if the user provides unexpected input, such as a negative age. Without error handling, the program might crash. Here's an improved version of the OOP example incorporating exception handling:
public class BirthdayGreeting {
// ... (previous code) ...
public void printGreeting() {
if (age < 0) {
System.out.println("Error: Age cannot be negative.");
return; // Exit the method gracefully
}
System.out.println("Happy Birthday, " + name + "! You are " + age + " years old.");
}
// ... (main method) ...
}
This demonstrates a simple form of exception handling. For more complex scenarios, using try-catch
blocks would be appropriate.
Further Analysis of "Error Handling"
Error handling is crucial for creating reliable software. Ignoring potential errors can lead to program crashes, data corruption, or unexpected behavior. Effective error handling involves:
- Anticipating Errors: Identifying potential sources of errors (e.g., invalid input, file not found).
- Implementing Error Checks: Adding code to detect errors before they cause problems.
- Handling Errors Gracefully: Providing informative error messages and taking appropriate corrective actions.
- Logging Errors: Recording error details for debugging and analysis.
Error Type | Description | Mitigation Strategy |
---|---|---|
Invalid Input | Incorrect data types or values provided by the user. | Input validation, data type checking, exception handling. |
File Not Found | Attempting to access a file that does not exist. | Check file existence, handle FileNotFoundException. |
Network Errors | Problems communicating with a network resource. | Handle network exceptions, retry mechanisms. |
OutOfMemoryError | Insufficient memory to run the program. | Optimize memory usage, increase heap size. |
NullPointerException | Accessing a null object reference. | Null checks before accessing object members. |
FAQ Section
-
Q: Can I use Java to create interactive birthday greetings? A: Yes! Java's Swing or JavaFX libraries allow creation of graphical user interfaces (GUIs) for interactive greetings.
-
Q: How can I add images or sound to my birthday greetings? A: Using Java's multimedia capabilities, you can incorporate images and sounds to enhance the greetings.
-
Q: Is there a way to send the birthday message via email? A: Yes, you can use JavaMail API to send email notifications.
-
Q: How can I personalize the birthday message further? A: You can incorporate user input, such as preferred greetings or inside jokes.
-
Q: Are there any online resources for learning more about Java? A: Many excellent online tutorials, courses, and documentation exist.
-
Q: Can I use external libraries to make the birthday message more advanced? A: Absolutely! Java's extensive library ecosystem allows integration of various functionalities.
Practical Tips
- Start Simple: Begin with basic string concatenation and gradually add complexity.
- Use Variables: Store data in variables for better code organization and reusability.
- Employ Conditional Statements: Personalize the message based on user input or specific conditions.
- Incorporate Loops: Repeat actions for multiple users or occasions.
- Create Reusable Methods: Encapsulate code blocks for better organization and maintainability.
- Use Objects: Represent data and behavior using classes and objects.
- Handle Errors: Anticipate potential errors and implement appropriate handling mechanisms.
- Test Thoroughly: Test the code with different inputs and scenarios to ensure correctness.
Final Conclusion
Saying "Happy Birthday" in Java is far more than a simple programming exercise. It's a powerful demonstration of core programming principles. This comprehensive guide has shown how even a seemingly basic task can be used to explore data types, control flow, object-oriented programming, input/output, and error handling. The examples and explanations provided equip you not only to create birthday messages but also to tackle more complex programming challenges. The journey of creating a simple greeting in Java highlights the depth and versatility of the language, highlighting its ability to adapt to diverse programming needs. This is just the beginning of what you can accomplish with Java. Go forth and create!
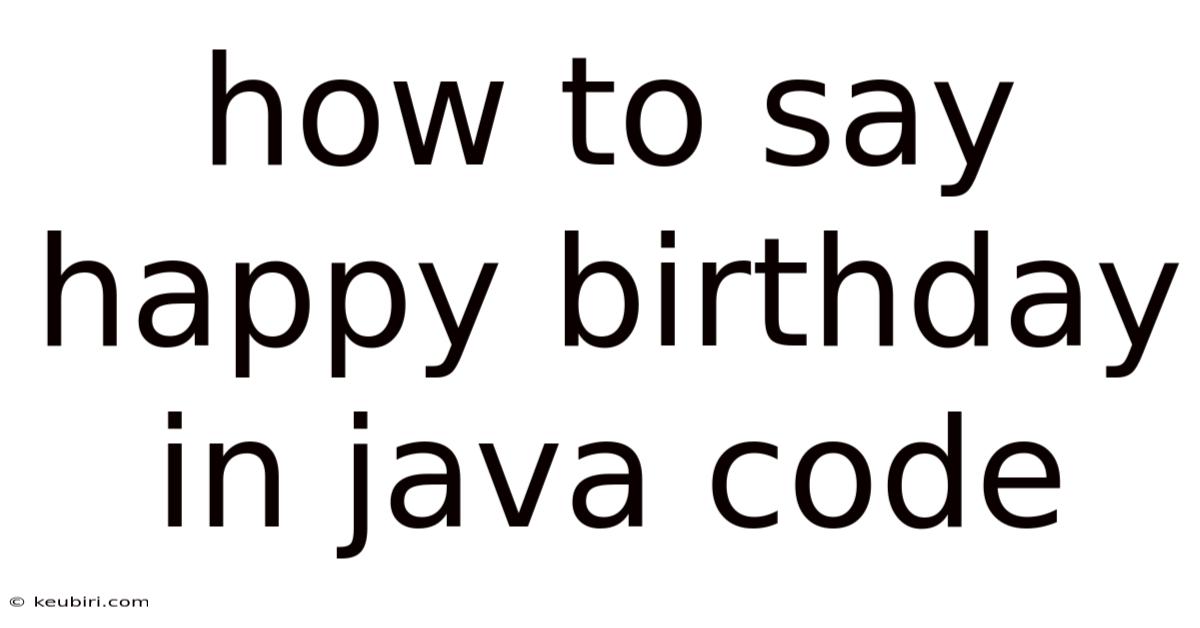
Thank you for visiting our website wich cover about How To Say Happy Birthday In Java Code. We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and dont miss to bookmark.
Also read the following articles
Article Title | Date |
---|---|
How To Say Wind In German | Apr 19, 2025 |
How To Say Mike In Chinese | Apr 19, 2025 |
How To Say Take Me Back In Spanish | Apr 19, 2025 |
How To Say Stop Playing In Spanish | Apr 19, 2025 |
How To Say Well Done To A Colleague | Apr 19, 2025 |