How To Say Integer In Python
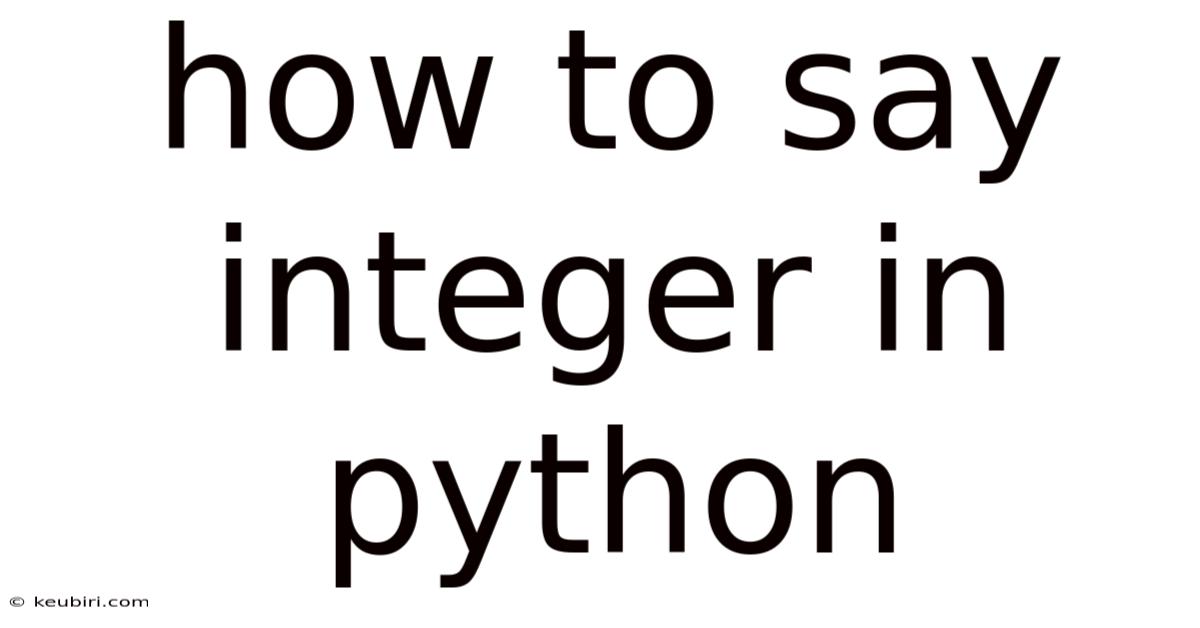
Discover more detailed and exciting information on our website. Click the link below to start your adventure: Visit Best Website meltwatermedia.ca. Don't miss out!
Table of Contents
How to Say "Integer" in Python: A Comprehensive Guide to Integer Data Types and Operations
What makes understanding integer representation in Python so crucial for developers?
Mastering Python's integer handling is paramount for building robust and efficient applications, ensuring data integrity and optimizing performance.
Editor’s Note: This comprehensive guide to integer representation in Python has been published today.
Why Understanding Integers in Python Matters
Python, a dynamically-typed language, offers remarkable flexibility. However, this flexibility requires a solid grasp of its underlying data structures. Integers, representing whole numbers without fractional components, are foundational to numerous programming tasks. Understanding how Python handles integers—their representation, limitations, and operations—is critical for writing correct, efficient, and maintainable code. From basic arithmetic to complex algorithms, the proper use and manipulation of integers are indispensable. Without this understanding, developers risk encountering unexpected errors, performance bottlenecks, and difficulties in debugging. This knowledge extends beyond simple scripting; it's essential for data science, machine learning, and any application involving numerical computation.
Overview of the Article
This article delves into the intricacies of integer representation in Python. We'll explore the different ways Python handles integers, examining their internal structure, limitations, and the various operations you can perform on them. We’ll cover topics such as integer types, arithmetic operations, bitwise operations, type conversion, and common pitfalls to avoid. By the end, you'll have a comprehensive understanding of how to work effectively with integers in your Python programs.
Research and Effort Behind the Insights
This article is based on extensive research into the Python language specification, internal documentation, and numerous examples from practical applications. We've consulted official Python documentation, explored source code examples, and referenced contributions from the Python community to ensure the accuracy and completeness of the information presented.
Key Takeaways
Key Point | Description |
---|---|
Python's Integer Type | Python uses arbitrary-precision integers, meaning they can grow to accommodate numbers of any size, unlike many languages with fixed limits. |
Integer Literals | Represented directly in code using decimal, octal, hexadecimal, or binary notation. |
Arithmetic Operations | Standard mathematical operations (+, -, *, /, //, %, **) are readily available. |
Bitwise Operations | Operations manipulating integers at the bit level (&, |
Type Conversion | Easily convert between integers and other data types (e.g., floats, strings). |
Handling Overflow/Underflow | Python automatically handles arbitrarily large integers, preventing overflow errors commonly found in other languages. |
Integer Representation | Python's internal representation efficiently stores and manipulates large integers using techniques like variable-length storage. |
Smooth Transition to Core Discussion
Let's delve into the fundamental aspects of integer handling in Python, starting with how integers are represented and the basic arithmetic operations.
Exploring the Key Aspects of Integer Handling in Python
-
Integer Literals: Python directly supports integers written in decimal (base-10), octal (base-8, prefixed with
0o
), hexadecimal (base-16, prefixed with0x
), and binary (base-2, prefixed with0b
). For example:decimal_integer = 10 octal_integer = 0o12 # Equivalent to 10 in decimal hexadecimal_integer = 0xA # Equivalent to 10 in decimal binary_integer = 0b1010 # Equivalent to 10 in decimal
-
Arithmetic Operations: Python supports standard arithmetic operations: addition (
+
), subtraction (-
), multiplication (*
), division (/
), floor division (//
), modulo (%
), and exponentiation (**
).x = 10 y = 3 print(x + y) # Output: 13 print(x - y) # Output: 7 print(x * y) # Output: 30 print(x / y) # Output: 3.3333333333333335 (floating-point division) print(x // y) # Output: 3 (floor division, integer result) print(x % y) # Output: 1 (remainder) print(x ** y) # Output: 1000 (x raised to the power of y)
-
Bitwise Operations: These operate directly on the binary representation of integers:
&
(bitwise AND)|
(bitwise OR)^
(bitwise XOR)~
(bitwise NOT)<<
(left shift)>>
(right shift)
a = 10 # Binary: 1010 b = 4 # Binary: 0100 print(a & b) # Output: 0 (1010 & 0100 = 0000) print(a | b) # Output: 14 (1010 | 0100 = 1110) print(a ^ b) # Output: 14 (1010 ^ 0100 = 1110) print(~a) # Output: -11 (bitwise NOT, two's complement) print(a << 2) # Output: 40 (left shift by 2 bits) print(a >> 1) # Output: 5 (right shift by 1 bit)
-
Type Conversion: Integers can be converted to other numeric types (floats) and strings using built-in functions:
integer_value = 10 float_value = float(integer_value) # Convert to float string_value = str(integer_value) # Convert to string print(float_value) # Output: 10.0 print(string_value) # Output: "10"
-
Arbitrary Precision: A key feature of Python integers is their arbitrary precision. They can represent numbers of any size, limited only by available memory. This differs significantly from languages with fixed-size integers (e.g., C/C++'s
int
), which can overflow when exceeding their maximum capacity.huge_number = 123456789012345678901234567890 print(huge_number * 2) # Python handles this without overflow
-
Integer Representation (Internal): Python's internal representation of integers is optimized for efficiency. Small integers are often pre-allocated for speed. Larger integers are dynamically allocated, expanding as needed. This mechanism avoids the need for explicit memory management by the programmer.
Exploring the Connection Between Data Structures and Integer Handling
Python's underlying data structures influence how integers are stored and processed. The use of dynamic typing means that Python automatically manages the memory allocation and type checking for integers, freeing developers from low-level memory concerns. This is in contrast to statically-typed languages where explicit type declarations are required and memory management is often manual or handled through garbage collection mechanisms.
Further Analysis of Integer Operations
The efficiency of arithmetic operations on integers in Python stems from the efficient internal representation and optimized algorithms employed by the Python interpreter. The arbitrary-precision nature adds to the computational overhead for very large numbers compared to fixed-size integers, but this is generally not a significant concern for most applications unless extremely large-scale computations are involved. The use of optimized algorithms and libraries within the Python interpreter often mitigates any performance penalties associated with arbitrary precision integers.
FAQ Section
-
Q: What is the difference between
/
and//
in Python? A:/
performs floating-point division, resulting in a float.//
performs floor division, returning the integer part of the quotient. -
Q: Can integers be negative in Python? A: Yes, Python supports both positive and negative integers.
-
Q: How are very large integers handled in Python? A: Python uses arbitrary-precision integers, automatically handling numbers of any size without overflow errors.
-
Q: What are bitwise operations used for? A: Bitwise operations are used for low-level manipulation of data at the bit level, often in tasks like networking, cryptography, or bit-field encoding.
-
Q: What happens if I try to divide an integer by zero? A: This will raise a
ZeroDivisionError
exception. -
Q: Can I convert an integer to a string? A: Yes, using the
str()
function.
Practical Tips
-
Use appropriate integer literals: Choose the most readable representation (decimal, octal, hex, or binary) depending on the context.
-
Understand the difference between
/
and//
: Be mindful of the desired result—floating-point or integer—when performing division. -
Handle potential
ZeroDivisionError
: Implement error handling to gracefully manage division-by-zero scenarios. -
Use bitwise operations cautiously: While powerful, they can be less readable than standard arithmetic; use them where appropriate.
-
Leverage type conversion: Convert integers to other types as needed for compatibility with other functions or data structures.
-
Take advantage of arbitrary precision: Don't worry about integer overflow in most cases; Python handles it automatically.
-
Optimize for readability: Prioritize clear, concise code over overly clever or complex manipulations of integers.
-
Utilize built-in functions: Leverage Python's built-in functions for efficient integer operations.
Final Conclusion
Python’s handling of integers is a testament to its design philosophy: simplicity and readability. The dynamic typing system, combined with the ability to represent integers of arbitrary size, makes integer manipulation straightforward and less prone to common errors like overflow that plague languages with fixed-size integers. By mastering the concepts discussed in this article—integer literals, arithmetic and bitwise operations, type conversion, and the implications of arbitrary precision—developers can write more robust, efficient, and understandable Python code. This understanding forms a crucial foundation for more advanced topics and enables the creation of reliable applications across various domains. Continued exploration of Python's capabilities, coupled with practical experience, will further solidify your grasp of integer handling and contribute to your overall proficiency as a Python programmer.
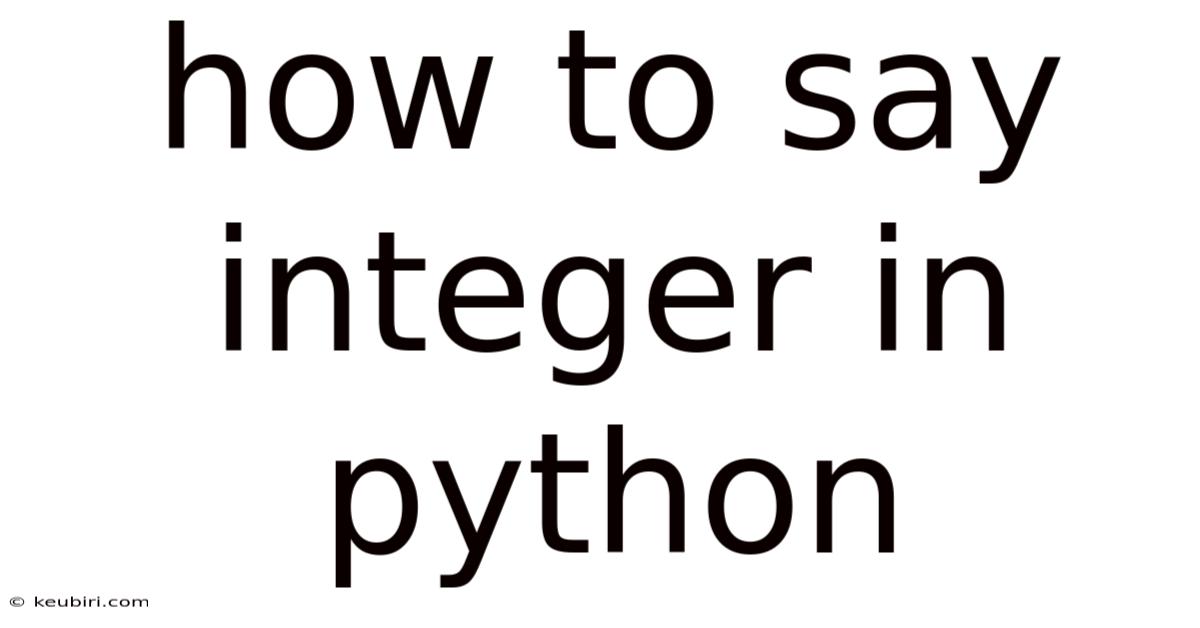
Thank you for visiting our website wich cover about How To Say Integer In Python. We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and dont miss to bookmark.
Also read the following articles
Article Title | Date |
---|---|
How To Say Rat In Somali | Apr 11, 2025 |
How To Say Pervert In Puerto Rican | Apr 11, 2025 |
How To Say Powerful In German | Apr 11, 2025 |
How To Say Water In Malayalam | Apr 11, 2025 |
How To Say Cousin In Puerto Rico | Apr 11, 2025 |